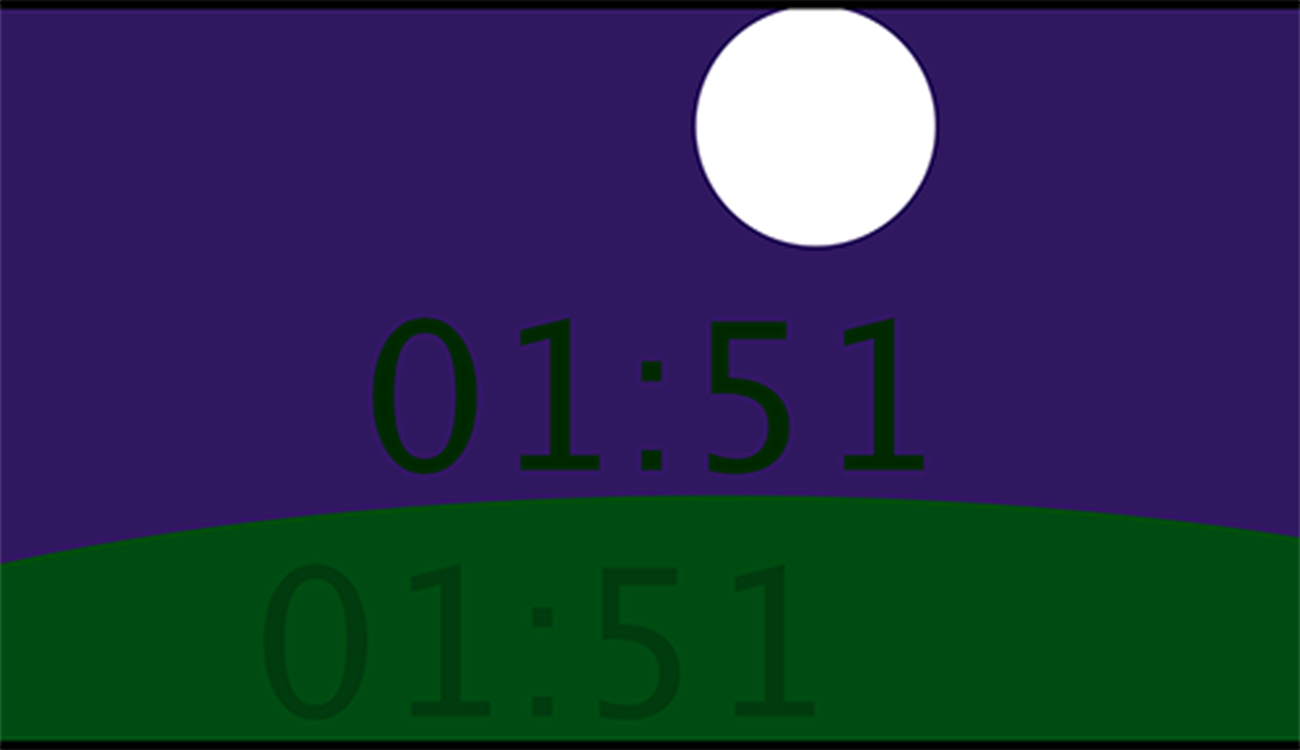
Creative Coding: Shop Clock
Creative Coding: Shop Clock
The latest assignment in my Critical Making class was to use Processing to create a clock for our studio room. We were tasked to be creative and think outside the clock. We just needed to represent time in a visual way. I chose to simulate the sun and moon moving across the sky with each hour. This was difficult for me and in the end I kind of cheated. But the result is the same.
Clock V1
The first version was not so much a clock as it was the background for the clock.
Here is the code:
int s = second();
int m = minute();
int h = hour();
void setup() {
//size(1280,800);
//pixelDensity(2);
//photo = loadImage("SunnyAfternoon.jpg");
fullScreen();
background(135,206,235);
println(width);
println(height);
}
void draw() {
fill(1,166,17);
noStroke();
arc(720, 900, 1450, 450, PI, 2*PI);
fill(249,250,87);
ellipse(150,150,200,200);
stroke(0);
line(300,300,350,350);
line(350,350,400,300);
line(500,500,550,550);
line(550,550,600,500);
line(800,100,850,150);
line(850,150,900,100);
}
Clock V1.1
This was a superior background with the grass and birds in a better position. I also divided height and width up so that the program would work on different screen sizes.
Here is the code:
float m = minute();
float h = hour();
float x;
float y;
float h1;
float w;
float x1 = (map(hour(),7,19,7,width));
float y1 = (map(hour(),7,19,7,height));
void setup() {
//size(1280,800);
//pixelDensity(2);
//photo = loadImage("SunnyAfternoon.jpg");
fullScreen();
background(135,206,235);
x = 0;
y = 0;
h1 = height/24;
w = width/12;
println(width);
println(height);
}
void draw() {
fill(1,166,17);
noStroke();
arc(x+(w*6+10), y+h1*25, x+(w*13+10), y+h1*12, PI, 2*PI);
fill(249,250,87);
ellipse(x1,y1+12,150,150);
stroke(0);
line(300,300,350,350);
line(350,350,400,300);
line(500,500,550,550);
line(550,550,600,500);
line(800,100,850,150);
line(850,150,900,100);
}
Clock V1.2
It was slow going trying to get the ellipse that makes up the sun and moon to be in the correct shape and position. I also began to attempt to map hours to position but was quite a ways off.
Here is the code:
float m = minute();
float h = hour();
float x;
float y;
float h1;
float w;
float x1 = (map(hour(),7,19,7,w));
float y1 = (map(hour(),7,19,7,h));
void setup() {
//size(1280,800);
//pixelDensity(2);
//photo = loadImage("SunnyAfternoon.jpg");
fullScreen();
background(135,206,235);
x = 0;
y = 0;
h1 = height/24;
w = width/12;
println(width);
println(height);
}
void draw() {
fill(1,166,17);
noStroke();
arc(x+(w*6+10), y+h1*25, x+(w*13+10), y+h1*12, PI, 2*PI);
fill(249,250,87);
ellipse(x1,y1+12,w,h*5);
stroke(0);
line(300,300,350,350);
line(350,350,400,300);
line(500,500,550,550);
line(550,550,600,500);
line(800,100,850,150);
line(850,150,900,100);
}
Clock V1.3
Finally, it’s a clock! I decided to opt for a 24 hour clock, something a little different. I still needed add a 0 before the hours and minutes when they are less than 10 so it follows the proper format 00:00. I divided height and width up in 24 segments. This gave me the partitions I needed to position everything. That ellipse though. Someday it’ll be in the right area…Someday.
Here is the code:
int m = minute();
int h = hour();
float x;
float y;
float h1;
float w;
void setup() {
//size(1280,800);
//pixelDensity(2);
//photo = loadImage("SunnyAfternoon.jpg");
fullScreen();
background(135,206,235);
x = 0;
y = 0;
h1 = height/24;
w = width/24;
}
void draw() {
//Grass, & sun/moon
fill(1,166,17);
noStroke();
arc(x+(w*12+10), y+h1*25, x+(w*25), y+h1*12, PI, 2*PI);
fill(249,250,87);
ellipse(x+w*4,y+h1*4,w*4,h*10);
String om = "";
if(m<10) om = "0";
else om = "";
//Clock
String t = h+":"+om+m;
textSize(250);
text(t,x+w*5,y+h1*8,x+w*15,y+h1*15);
}
Clock V1.4
In this version I added a couple additional elements. A new string with some logic that put a 0 in front of minutes if they were less than 10. I changed the size of the arc and added a shadow. I started experimenting with the position of the clock and shadow. I got the ellipse in the right spot but it is hardly a sun or moon shape. That bleeping ellipse.
Here is the code:
int m = minute();
int h = hour();
float x;
float y;
float h1;
float w;
void setup() {
//size(1280,800);
//pixelDensity(2);
//photo = loadImage("SunnyAfternoon.jpg");
fullScreen();
background(135,206,235);
x = 0;
y = 0;
h1 = height/24;
w = width/24;
}
void draw() {
//Grass, & sun/moon
fill(1,166,17);
noStroke();
arc(x+(w*12+10), y+h1*25, x+(w*25), y+h1*18, PI, 2*PI);
fill(249,250,87);
ellipse(x+w*4,y+h1*4,w*4,h*10);
String om = "";
if(m<10) om = "0";
else om = "";
//Clock
String t = h+":"+om+m;
textSize(250);
fill(255,255,255);
text(t,x+w*6,y+h1*8,x+w*15,y+h1*15);
//Shadow
textSize(250);
fill(0);
text(t,x+w*10,y+h1*17.5,x+w*15,y+h1*17.5);
}
Clock V1.5
I decided to center the ellipse, text, and shadow as if it was noon or midnight. I began to experiment with if logic, if poorly at first in an attempt to change the color for night time.
Here is the code:
int m = minute();
int h = hour();
int s = second();
float x;
float y;
float h1;
float w;
void setup() {
fullScreen();
if (h>17 && h<7) background (48,24,96); //Make background night sky color
else background(135,206,235);
//background(135,206,235);
x = 0;
y = 0;
h1 = height/24;
w = width/24;
println (width);
println (height);
}
void draw() {
//Grass, & sun/moon
if (h>17 && h<7) fill (0,75,17); //change arc color for night.
else fill(1,166,17);
noStroke();
arc(x+(w*12+10), y+h1*25, x+(w*25), y+h1*18, PI, 2*PI);
//if (h>6 && h<11) fill
if (h>17 && h<7) fill (255,255,255); //turns sun to moon.
else fill (249,250,87);
ellipse(x+w*12,y+h1*4,w*4,h*16); //need to add if so move sun to left side before noon and right after noon
String om = "";
if(m<10) om = "0";
else om = "";
//Clock
String t = h + ":" + om + m;
textSize(250);
if (h>17 && h<7) fill (192,192,192); //Changes Clock from white to silver for night
else fill(255,255,255);
text(t,x+w*7.5,y+h1*8,x+w*15,y+h1*15);
//Shadow
textSize(250);
if (h>17 && h<7) fill(255,255,255); //changes shadow from black to white
else fill(0);
text(t,x+w*7.5,y+h1*17.5,x+w*15,y+h1*17.5);
}
Clock V1.6
Whoa, it’s a sphere! All I had to do was set the ellipse’s height and width to constants. Duh. And the text is perfectly centered after learning about the textAlign command. The if logic is also not working, making it night time.
Here is the code:
float x;
float y;
float h1;
float w;
float st;
void setup() {
fullScreen();
x = 0;
y = 0;
h1 = height/24;
w = width/24;
println (width);
println (height);
}
void draw() {
int m = minute();
int h = hour();
int s = second();
if ((h>17) || (h<7)) { //Make background night sky color
background (48,24,96);
}
else {
background(135,206,235);
}
//Grass, & sun/moon
if ((h>17) || (h<7)) { //change arc color for night.
fill (0,75,17);
}
else {
fill(1,166,17);
}
noStroke();
arc(x+(w*12+10), y+h1*25, x+(w*25), y+h1*18, PI, 2*PI);
if ((h>17) || (h<7)) {
fill (255,255,255); //turns sun to moon.
}
else {
fill (249,250,87);
}
ellipse((x+w*1),(y+h1*12),250,250); //need to add if so move sun to left side before noon and right after noon
st = st + (w*2);
String om = "";
if(m<10) {
om = "0";
}
else {
om = "";
}
//Clock
String t = h + ":" + om + m;
textSize(250);
if ((h>17) || (h<7)) {
fill (192,192,192);
} //Changes Clock from white to silver for night
else {
fill(255,255,255);
}
textAlign(CENTER,CENTER);
text(t, x+(w*12), y+(h1*12));
//Shadow
textSize(250);
textAlign(CENTER,CENTER);
fill(0);
text(t, x+w*12, y+h1*20);
}
Clock V1.7
Version 1.7 had a lot of different attempts. With the help of a classmate, I was able to get the ellipse to rotate around the screen from the middle in the correct direction an mapped to seconds. But for the life of me I could not figure out how to set the intervals the ellipse moved, nor could I figure out how to reset to the left ‘horizon’ every 12 hours. I spent hours trying to figure this out but eventually I opted to try a different route. Specifically, I decided to use If logic to move the ellipse depending on the hour. It didn’t exactly work out at first. I did get a 0 in front of hours though.
Here is the code:
float x;
float y;
float h1; //height
float w;
//float x1;
//float y1;
void setup() {
fullScreen();
x = 0;
y = 0;
h1 = height/24;
w = width/24;
println (width);
println (height);
}
void draw() {
int m = minute();
int h = hour();
int s = second();
if ((h>17) || (h<7)) { //Make background night sky color
background (48, 24, 96);
} else {
background(135, 206, 235);
}
//Grass, & sun/moon
if ((h>17) || (h<7)) { //change arc color for night.
fill (0, 75, 17);
} else {
fill(1, 166, 17);
}
noStroke();
arc(x+(w*12+10), y+h1*25, x+(w*25), y+h1*18, PI, 2*PI);
//Sun/Moon & rotation
//float x1;
//float y1;
//float x1 = map(second(), 15, 45, PI, 0)+PI;
//float y1 = map(second(), 15, 45, PI, 0)+PI;
//x1 = cos(s)*(w*12)+width/2-PI;
//y1 = sin(s)*(h1*12)+(h1*12)-PI;
if ((h>17) || (h<7)) {
fill (255, 255, 255); //turns sun to moon.
} else {
fill (249, 250, 87);
}
if ((h>6) || (h<12)) {
ellipse (x+w*6, y+h1*6, 250, 250);
}
if ((h>19) || (h<24)) {
ellipse (x+w*6, y+h1*6, 250, 250);
}
//if ((h>11) || (h<13)) {
// ellipse (x+w*12, y+h1*4, 250, 250);
// }
//if ((h>=0) || (h<4)) {
// ellipse (x+w*12, y+h1*4, 250, 250);
// }
if ((h>15) || (h<120)) {
ellipse (x+w*18, y+h1*6, 250, 250);
}
if ((h>3) || (h<7)) {
ellipse (x+w*18, y+h1*6, 250, 250);
} else {
ellipse (x+w*12, y+h1*4, 250, 250);
}
// Adds a 0 to minutes & hours when less than 10.
String om = "";
if (m<10) {
om = "0";
} else {
om = "";
}
String oh = "";
if (h<10) {
oh = "0";
} else {
oh = "";
}
//Clock
String t = oh + h + ":" + om + m;
textSize(250);
if ((h>17) || (h<7)) {
fill (192, 192, 192);
} //Changes Clock from white to silver for night
else {
fill(255, 255, 255);
}
textAlign(CENTER, CENTER);
text(t, x+(w*12), y+(h1*12));
//Shadow
textSize(250);
textAlign(CENTER, CENTER);
fill(0);
text(t, x+w*12, y+h1*20);
}
–
Clock V1.8
In the previous version I was just drawing a new ellipse. In this version I used If and Translate to move the ellipse. I did it for just three parts of the day and night. It all worked but was too simple. I wanted the sun and moon to move every hour.
float x;
float y;
float h1; //height
float w;
//float x1;
//float y1;
void setup() {
fullScreen();
x = 0;
y = 0;
h1 = height/24;
w = width/24;
println (width);
println (height);
}
void draw() {
int m = minute();
int h = hour();
int s = second();
if ((h>17) || (h<7)) { //Make background night sky color
background (48, 24, 96);
} else {
background(135, 206, 235);
}
// Adds a 0 to minutes & hours when less than 10.
String om = "";
if (m<10) {
om = "0";
} else {
om = "";
}
String oh = "";
if (h<10) {
oh = "0";
} else {
oh = "";
}
//Clock
String t = oh + h + ":" + om + m;
textSize(250);
if ((h>17) || (h<7)) { //Changes Clock from white to silver for night
fill (192, 192, 192);
}
else {
fill(255, 255, 255);
}
textAlign(CENTER, CENTER);
text(t, x+(w*12), y+(h1*12));
//Grass
if ((h>17) || (h<7)) { //change arc color for night.
fill (0, 75, 17);
} else {
fill(1, 166, 17);
}
noStroke();
arc(x+(w*12+10), y+h1*25, x+(w*25), y+h1*18, PI, 2*PI);
//Shadow
textSize(250);
textAlign(CENTER, CENTER);
if ((h>6) && (h<12) || (h>19 && h<24)) {
translate (x+w*8, y+0);
}
if ((h>3) && (h<7) || (h>15) && (h<20)) {
translate (x-w*8, y+0);
}
fill(0);
text(t, x+w*12, y+h1*20);
//Sun/Moon & rotation
//float x1;
//float y1;
//float x1 = map(second(), 15, 45, TWO_PI, 0)+PI;
//float y1 = map(second(), 15, 45, TWO_PI, 0)+PI;
//x1 = cos(s)*(w*12)+width/2-PI;
//y1 = sin(s)*(h1*12)+(h1*12)-PI;
if ((h>17) || (h<7)) {
fill (255, 255, 255); //turns sun to moon.
} else {
fill (249, 250, 87);
}
// ellipse (x+w*12, y+h1*4, 250, 250);
// ellipse (x+w*20, y+h1*8, 250, 250);
if ((h>11) || (h<16) && (h>=0 || h<4)) {
translate (x+w*8, y-h1*4);
}
if ((h>3) || (h<7) && (h>15) || (h<20)) {
translate (CENTER, y+0);
}
ellipse (x+w*4, y+h1*8, 250, 250);
}
Clock V1.9:Final Version
Lots of additions in the final version. First and foremost, I added an If and Translate for every hour so the sun and moon moved every hour. I increased the size of the ellipse and text and I have the clock some color, mapped to seconds so the user had an idea of how far they were into the minute.
Color change:
Clock Color Changes from Jim Murphy on Vimeo.
Here is the code:
float x;
float y;
float h1; //height
float w;
int xPos=200;
int yPos=200;
int xPos1=200;
int yPos1=200;
int storedMinute;
int minute;
void setup() {
fullScreen();
frameRate(30);
x = 0;
y = 0;
h1 = height/24;
w = width/24;
println (width);
println (height);
}
void draw() {
int m = minute();
int h = hour();
int s = second();
minute = minute();
if ((h>17) || (h<7)) { //Make background night sky color
background (48, 24, 96);
} else {
background(135, 206, 235);
}
// Adds a 0 to minutes & hours when less than 10.
String om = "";
if (m<10) {
om = "0";
} else {
om = "";
}
String oh = "";
if (h<10) {
oh = "0";
} else {
oh = "";
}
//Clock
float val = map(second(), 0, 60, 0, 127.5);
float val1 = map(second(), 0, 60, 127.5, 255);
String t = oh + h + ":" + om + m;
textSize(300);
textAlign(CENTER, CENTER);
if (storedMinute != minute()) {
fill(0);
}
if ((h>7) || (h<19)) {
fill(0, val1, 0);
}
fill(0, val, 0);
text(t, x+(w*12), y+(h1*12));
//Grass
if ((h>7) || (h<19)) {
fill(1, 170, 17);
} //change arc color for night.
fill (0, 75, 17);
noStroke();
arc(x+(w*12+100), y+h1*25, x+(w*40), y+h1*18, PI, 2*PI);
//Shadow
textSize(300);
textAlign(CENTER, CENTER);
fill(0, 0, 0, 50);
pushMatrix();
if ((h==8) || (h==20)) {
translate (x+w*.9, y+0);
}
if ((h==9) || (h==21)) {
translate (x-w*1.9, y+0);
}
if ((h==10) || (h==22)) {
translate (x-w*3.9, y+0);
}
if ((h==11) || (h==23)) {
translate (x-w*5.9, y+0);
}
if ((h==12) || (h==0)) {
translate (x-w*7.9, y+0);
}
if ((h==13) || (h==1)) {
translate (x-w*9.9, y+0);
}
if ((h==14) || (h==2)) {
translate (x-w*11.9, y+0);
}
if ((h==15) || (h==3)) {
translate (x-w*13.9, y+0);
}
if ((h==16) || (h==4)) {
translate (x-w*15.9, y+0);
}
if ((h==17) || (h==5)) {
translate (x-w*17.9, y+0);
}
if ((h==18) || (h==6)) {
translate (x-w*19.9, y+0);
}
text(t, x+w*19.9, y+h1*20);
popMatrix();
// Sun/Moon
if ((h>17) || (h<7)) {
fill (255, 255, 255); //turns sun to moon.
} else {
fill (249, 250, 87);
}
noStroke();
pushMatrix();
if ((h==8) || (h==20)) {
translate (x+w*3, y-h1*2);
}
if ((h==9) || (h==21)) {
translate (x+w*5, y-h1*4);
}
if ((h==10) || (h==22)) {
translate (x+w*7, y-h1*6);
}
if ((h==11) || (h==23)) {
translate (x+w*9, y-h1*8);
}
if ((h==12) || (h==0)) {
translate (x+w*12, y-h1*9);
}
if ((h==13) || (h==1)) {
translate (x+w*15, y-h1*8);
}
if ((h==14) || (h==2)) {
translate (x+w*17, y-h1*6);
}
if ((h==15) || (h==3)) {
translate (x+w*19, y-h1*4);
}
if ((h==16) || (h==4)) {
translate (x+w*21, y-h1*2);
}
if ((h==17) || (h==5)) {
translate (x+w*22, y-h1*1);
}
if ((h==18) || (h==6)) {
translate (x+w*23, y+0);
}
ellipse (x, y+h1*12, 350, 350);
popMatrix();
storedMinute = minute;
}
Summary
As I mentioned, this assignment was difficult. The amount of trigonometry required made my brain steam a bit. Drawing some things out was very helpful.
With some help from my professor, I learned how to use sin & cos, how to use radians, and how to map time to radians. Being able to directly apply the math to something visual helps me learn it much faster than how it was abstractly taught in school.
Some additions to the clock, I would like to use a greater color range in the clock fill and add some more elements to the background. But for now I am very happy with a clock that works and does what I want.